Here is some code that will help you create all the RGB values needed for generating a RGB Rainbow gradient. The code steps through 6 sets of transitions from one color to another in 256 steps each .
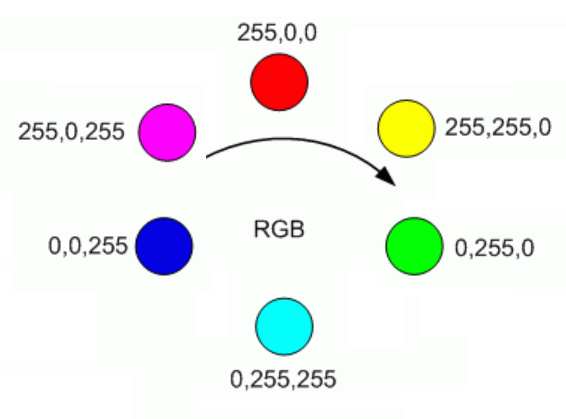
- Red to Yellow
- Yellow to Green
- Green to Cyan
- Cyan to Blue
- Blue to Magenta
- Magenta back to Red
With 6 transitions, each in 256 steps provides 1536 total colors and here is the result from the code below:
See it in action here: https://codepen.io/alijani/pen/zYVmzqR
html file:
<canvas id="myCanvas" width="1536" height="200"></canvas>
Js File:
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
var x;
for (x=0; x<1536; x++) { // 6*256
ctx.fillStyle = rainbow(x);
ctx.fillRect(x, 0, x, 200);
}
// Accepts values 0 through 1535 and returns the one of 1536 colors in rainbow.
function rainbow(x) {
let r = Math.trunc(x / 256); // 0-5
let c = x % 256;
if (r==0) {
value = rgb(255,c,0); // red to yellow transition
} else if (r==1) {
value = rgb(255-c,255,0); // yellow to green transition
} else if (r==2) {
value = rgb(0,255,c); // green to cyan transition
} else if (r==3) {
value = rgb(0,255-c,255); // cyan to blue transition
} else if (r==4) {
value = rgb(c,0,255); // blue to magenta transition
} else if (r==5) {
value = rgb(255,0,255-c); // Magenta to ren transision
}
return value;
}
// simple function to convert rgb decimal values to #RRGGBB hex color string used in html
function rgb(r,g,b) {
return "#"+(r).toString(16).padStart(2,'0')+(g).toString(16).padStart(2,'0')+(b).toString(16).padStart(2,'0');
}
Leave a comment
Your email address will not be published. Required fields are marked *